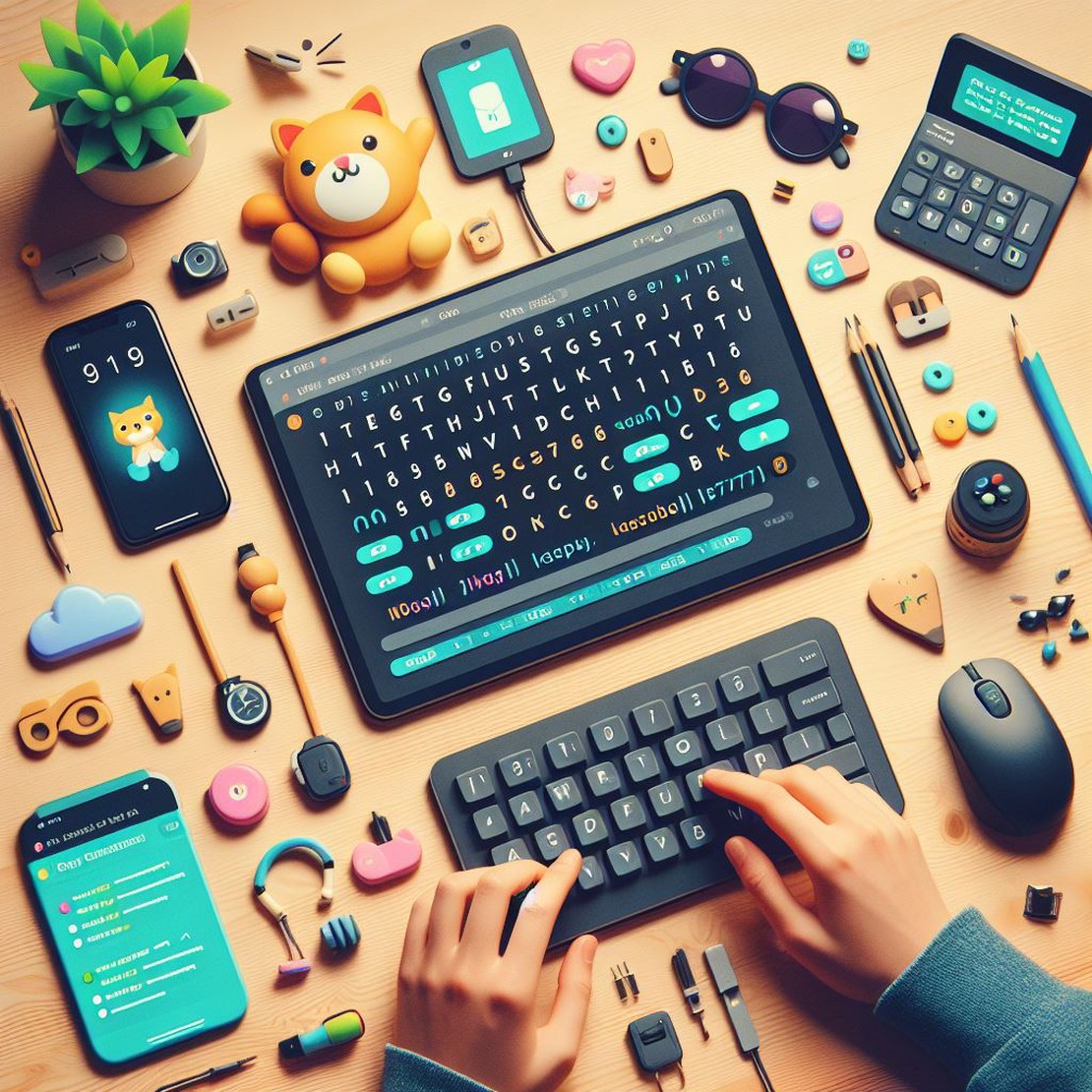
Creating a Morse Code Generator App in 10 Minutes!
- Rajdeep Barad
- Flutter , App development
- January 27, 2024
Morse code has a rich history and is a fascinating method of communication. Invented in the early 1800s by Samuel Morse and Alfred Vail, it was widely used in telegraphy before modern communication methods took over. Even today, Morse code still holds a special place in the hearts of amateur radio operators and enthusiasts.
In this blog post, you will learn how to create a Morse code generator app in just 10 minutes using the Flutter framework. Flutter allows you to build beautiful and performant cross-platform apps with ease. So let’s dive in!
Setting Up the Project
To get started, make sure you have Flutter installed on your machine. If you haven’t done so already, head over to the official Flutter website and follow the installation instructions for your operating system.
Once Flutter is set up, open your favorite code editor and create a new Flutter project. You can use the following command in your terminal:
flutter create morse_code_generator
This command will create a new Flutter project named morse_code_generator
. Navigate into the project directory by running:
cd morse_code_generator
Designing the User Interface
The next step is to design the user interface of our Morse code generator app. Open the lib/main.dart
file in your code editor.
Remove the default code and replace it with the following:
import 'package:flutter/material.dart';
void main() {
runApp(MorseCodeGeneratorApp());
}
class MorseCodeGeneratorApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Morse Code Generator',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MorseCodeGeneratorScreen(),
);
}
}
class MorseCodeGeneratorScreen extends StatefulWidget {
@override
_MorseCodeGeneratorScreenState createState() =>
_MorseCodeGeneratorScreenState();
}
class _MorseCodeGeneratorScreenState extends State<MorseCodeGeneratorScreen> {
TextEditingController _textEditingController = TextEditingController();
String _morseCodeResult = '';
@override
void dispose() {
_textEditingController.dispose();
super.dispose();
}
void _generateMorseCode() {
// Your morse code generation logic goes here
// Convert the text entered by the user to morse code
// Update the `_morseCodeResult` variable with the morse code result
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Morse Code Generator'),
),
body: Padding(
padding: EdgeInsets.all(16.0),
child: Column(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: [
TextField(
controller: _textEditingController,
decoration: InputDecoration(
labelText: 'Enter text',
),
),
SizedBox(height: 16.0),
ElevatedButton(
onPressed: _generateMorseCode,
child: Text('Generate Morse Code'),
),
SizedBox(height: 16.0),
Text(
'Morse Code Result:',
style: Theme.of(context).textTheme.headline6,
),
Text(_morseCodeResult),
],
),
),
);
}
}
This code sets up the basic structure of our app and provides a simple user interface with a text input field, a button to generate Morse code, and a text widget to display the generated Morse code.
Generating Morse Code
Next, let’s implement the _generateMorseCode
function to convert the text entered by the user to Morse code. Add the following code inside the _MorseCodeGeneratorScreenState
class:
void _generateMorseCode() {
String text = _textEditingController.text.toUpperCase();
String morseCode = '';
for (int i = 0; i < text.length; i++) {
String character = text[i];
if (character == 'A') {
morseCode += '.- ';
} else if (character == 'B') {
morseCode += '-... ';
} else if (character == 'C') {
morseCode += '-.-. ';
}
// Add Morse code mappings for other characters here
// ...
}
setState(() {
_morseCodeResult = morseCode.trim();
});
}
In this code, we convert the text entered by the user to uppercase and initialize an empty string variable morseCode
to store the generated Morse code. We then iterate over each character in the input text and append the corresponding Morse code representation to the morseCode
variable.
You can add Morse code mappings for other characters using if
statements or consider using a map for a more efficient implementation.
Finally, we update the _morseCodeResult
variable with the generated Morse code and call setState
to refresh the user interface and display the updated result.
Running the App
Congratulations! You have completed the app development. It’s time to run the app and see it in action. Make sure you have an Android or iOS device connected or an emulator running. Use the following command to launch the app:
flutter run
Flutter will build the app and launch it on your connected device or emulator. You will be able to enter text, generate Morse code, and see the result on the screen.
Conclusion
In just 10 minutes, you have created a Morse code generator app using Flutter. This simple app demonstrates the power and ease of Flutter for building cross-platform apps.
Feel free to enhance the app by adding more features such as sound playback for the Morse code or a reverse conversion from Morse code to text. Explore the rich Flutter ecosystem and experiment with different UI designs.
I hope you enjoyed this tutorial and discovered the fun and creativity behind building a Morse code generator app. Happy coding!
Ready to transform your digital vision?
Get in touch with us to explore how our cutting-edge solutions can elevate your business to new heights. Contact us today!
Get in Touch